1) Jocul Snake (rezolvare...)
2) Jocul Tetris (rezolvare...)
3) Jocul mini Tenis (rezolvare...)
Aplicatii Java: jocuri (codul sursa)
Rezolvari
Se face un proiect java, in Eclipse.
(vezi aici utilizare Eclipse pentru dezvoltarea aplicaţiilor Java)
Atentie! Numele clasei declarate public, coincide cu numele fisierului java (fara extensie)
In cadrul lui, se fac doua fisiere .java care se numesc Board si Snake in care se copiaza codul de mai jos:
(vezi aici utilizare Eclipse pentru dezvoltarea aplicaţiilor Java)
Atentie! Numele clasei declarate public, coincide cu numele fisierului java (fara extensie)
In cadrul lui, se fac doua fisiere .java care se numesc Board si Snake in care se copiaza codul de mai jos:
//Board.java import java.awt.Color; import java.awt.Font; import java.awt.FontMetrics; import java.awt.Graphics; import java.awt.Image; import java.awt.Toolkit; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.KeyAdapter; import java.awt.event.KeyEvent; import javax.swing.ImageIcon; import javax.swing.JPanel; import javax.swing.Timer; public class Board extends JPanel implements ActionListener { private static final long serialVersionUID = 1L; private final int WIDTH = 300; private final int HEIGHT = 300; private final int DOT_SIZE = 10; private final int ALL_DOTS = 900; private final int RAND_POS = 29; private final int DELAY = 140; private int x[] = new int[ALL_DOTS]; private int y[] = new int[ALL_DOTS]; private int dots; private int apple_x; private int apple_y; private boolean left = false; private boolean right = true; private boolean up = false; private boolean down = false; private boolean inGame = true; private Timer timer; private Image ball; private Image apple; private Image head; public Board() { addKeyListener(new TAdapter()); setBackground(Color.black); ImageIcon iid = new ImageIcon(this.getClass().getResource("dot.png")); ball = iid.getImage(); ImageIcon iia = new ImageIcon(this.getClass().getResource("apple.png")); apple = iia.getImage(); ImageIcon iih = new ImageIcon(this.getClass().getResource("head.png")); head = iih.getImage(); setFocusable(true); initGame(); } public void initGame() { dots = 3; for (int z = 0; z < dots; z++) { x[z] = 50 - z*10; y[z] = 50; } locateApple(); timer = new Timer(DELAY, this); timer.start(); } public void paint(Graphics g) { super.paint(g); if (inGame) { g.drawImage(apple, apple_x, apple_y, this); for (int z = 0; z < dots; z++) { if (z == 0) g.drawImage(head, x[z], y[z], this); else g.drawImage(ball, x[z], y[z], this); } Toolkit.getDefaultToolkit().sync(); g.dispose(); } else { gameOver(g); } } public void gameOver(Graphics g) { String msg = "Game Over"; Font small = new Font("Helvetica", Font.BOLD, 14); FontMetrics metr = this.getFontMetrics(small); g.setColor(Color.white); g.setFont(small); g.drawString(msg, (WIDTH - metr.stringWidth(msg)) / 2, HEIGHT / 2); } public void checkApple() { if ((x[0] == apple_x) && (y[0] == apple_y)) { dots++; locateApple(); } } public void move() { for (int z = dots; z > 0; z--) { x[z] = x[(z - 1)]; y[z] = y[(z - 1)]; } if (left) { x[0] -= DOT_SIZE; } if (right) { x[0] += DOT_SIZE; } if (up) { y[0] -= DOT_SIZE; } if (down) { y[0] += DOT_SIZE; } } public void checkCollision() { for (int z = dots; z > 0; z--) { if ((z > 4) && (x[0] == x[z]) && (y[0] == y[z])) { inGame = false; } } if (y[0] > HEIGHT) { inGame = false; } if (y[0] < 0) { inGame = false; } if (x[0] > WIDTH) { inGame = false; } if (x[0] < 0) { inGame = false; } } public void locateApple() { int r = (int) (Math.random() * RAND_POS); apple_x = ((r * DOT_SIZE)); r = (int) (Math.random() * RAND_POS); apple_y = ((r * DOT_SIZE)); } public void actionPerformed(ActionEvent e) { if (inGame) { checkApple(); checkCollision(); move(); } repaint(); } private class TAdapter extends KeyAdapter { public void keyPressed(KeyEvent e) { int key = e.getKeyCode(); if ((key == KeyEvent.VK_LEFT) && (!right)) { left = true; up = false; down = false; } if ((key == KeyEvent.VK_RIGHT) && (!left)) { right = true; up = false; down = false; } if ((key == KeyEvent.VK_UP) && (!down)) { up = true; right = false; left = false; } if ((key == KeyEvent.VK_DOWN) && (!up)) { down = true; right = false; left = false; } } } }
//Snake.java import javax.swing.JFrame; public class Snake extends JFrame { private static final long serialVersionUID = 1L; public Snake() { add(new Board()); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(320, 340); setLocationRelativeTo(null); setTitle("Snake"); setResizable(false); setVisible(true); } public static void main(String[] args) { new Snake(); } }
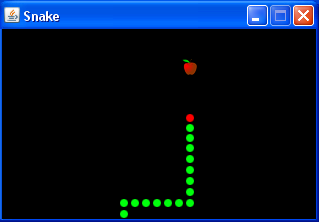
In C:\Documents and Settings\numecomputer\workspace\numeproiect\bin\
trebuiesc adaugate 3 poze cu extensia .png cu denumirile: dot, apple, head,
cu dimensiunea de 10X10.
Pentru a rula codul de mai sus, apasati butonul run din Eclipse si
vi se va deschide jocul, ca-n imaginea alaturata.
Pentru a downloada proiectul cu toate fisierele faceti click aici.
Se face un proiect java, in Eclipse.
(vezi aici utilizare Eclipse pentru dezvoltarea aplicaţiilor Java)
Atentie! Numele clasei declarate public, coincide cu numele fisierului java (fara extensie)
In cadrul lui, se fac trei fisiere .java care se numesc Board, Shape si Tetris in care se copiaza codul de mai jos:
(vezi aici utilizare Eclipse pentru dezvoltarea aplicaţiilor Java)
Atentie! Numele clasei declarate public, coincide cu numele fisierului java (fara extensie)
In cadrul lui, se fac trei fisiere .java care se numesc Board, Shape si Tetris in care se copiaza codul de mai jos:
//Board.java package tetris; import java.awt.Color; import java.awt.Dimension; import java.awt.Graphics; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.KeyAdapter; import java.awt.event.KeyEvent; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.Timer; import tetris.Shape.Tetrominoes; public class Board extends JPanel implements ActionListener { final int BoardWidth = 10; final int BoardHeight = 22; Timer timer; boolean isFallingFinished = false; boolean isStarted = false; boolean isPaused = false; int numLinesRemoved = 0; int curX = 0; int curY = 0; JLabel statusbar; Shape curPiece; Tetrominoes[] board; public Board(Tetris parent) { setFocusable(true); curPiece = new Shape(); timer = new Timer(400, this); timer.start(); statusbar = parent.getStatusBar(); board = new Tetrominoes[BoardWidth * BoardHeight]; addKeyListener(new TAdapter()); clearBoard(); } public void actionPerformed(ActionEvent e) { if (isFallingFinished) { isFallingFinished = false; newPiece(); } else { oneLineDown(); } } int squareWidth() { return (int) getSize().getWidth() / BoardWidth; } int squareHeight() { return (int) getSize().getHeight() / BoardHeight; } Tetrominoes shapeAt(int x, int y) { return board[(y * BoardWidth) + x]; } public void start() { if (isPaused) return; isStarted = true; isFallingFinished = false; numLinesRemoved = 0; clearBoard(); newPiece(); timer.start(); } private void pause() { if (!isStarted) return; isPaused = !isPaused; if (isPaused) { timer.stop(); statusbar.setText("paused"); } else { timer.start(); statusbar.setText(String.valueOf(numLinesRemoved)); } repaint(); } public void paint(Graphics g) { super.paint(g); Dimension size = getSize(); int boardTop = (int) size.getHeight() - BoardHeight * squareHeight(); for (int i = 0; i < BoardHeight; ++i) { for (int j = 0; j < BoardWidth; ++j) { Tetrominoes shape = shapeAt(j, BoardHeight - i - 1); if (shape != Tetrominoes.NoShape) drawSquare(g, 0 + j * squareWidth(), boardTop + i * squareHeight(), shape); } } if (curPiece.getShape() != Tetrominoes.NoShape) { for (int i = 0; i < 4; ++i) { int x = curX + curPiece.x(i); int y = curY - curPiece.y(i); drawSquare(g, 0 + x * squareWidth(), boardTop + (BoardHeight - y - 1) * squareHeight(), curPiece.getShape()); } } } private void dropDown() { int newY = curY; while (newY > 0) { if (!tryMove(curPiece, curX, newY - 1)) break; --newY; } pieceDropped(); } private void oneLineDown() { if (!tryMove(curPiece, curX, curY - 1)) pieceDropped(); } private void clearBoard() { for (int i = 0; i < BoardHeight * BoardWidth; ++i) board[i] = Tetrominoes.NoShape; } private void pieceDropped() { for (int i = 0; i < 4; ++i) { int x = curX + curPiece.x(i); int y = curY - curPiece.y(i); board[(y * BoardWidth) + x] = curPiece.getShape(); } removeFullLines(); if (!isFallingFinished) newPiece(); } private void newPiece() { curPiece.setRandomShape(); curX = BoardWidth / 2 + 1; curY = BoardHeight - 1 + curPiece.minY(); if (!tryMove(curPiece, curX, curY)) { curPiece.setShape(Tetrominoes.NoShape); timer.stop(); isStarted = false; statusbar.setText("game over"); } } private boolean tryMove(Shape newPiece, int newX, int newY) { for (int i = 0; i < 4; ++i) { int x = newX + newPiece.x(i); int y = newY - newPiece.y(i); if (x < 0 || x >= BoardWidth || y < 0 || y >= BoardHeight) return false; if (shapeAt(x, y) != Tetrominoes.NoShape) return false; } curPiece = newPiece; curX = newX; curY = newY; repaint(); return true; } private void removeFullLines() { int numFullLines = 0; for (int i = BoardHeight - 1; i >= 0; --i) { boolean lineIsFull = true; for (int j = 0; j < BoardWidth; ++j) { if (shapeAt(j, i) == Tetrominoes.NoShape) { lineIsFull = false; break; } } if (lineIsFull) { ++numFullLines; for (int k = i; k < BoardHeight - 1; ++k) { for (int j = 0; j < BoardWidth; ++j) board[(k * BoardWidth) + j] = shapeAt(j, k + 1); } } } if (numFullLines > 0) { numLinesRemoved += numFullLines; statusbar.setText(String.valueOf(numLinesRemoved)); isFallingFinished = true; curPiece.setShape(Tetrominoes.NoShape); repaint(); } } private void drawSquare(Graphics g, int x, int y, Tetrominoes shape) { Color colors[] = { new Color(0, 0, 0), new Color(204, 102, 102), new Color(102, 204, 102), new Color(102, 102, 204), new Color(204, 204, 102), new Color(204, 102, 204), new Color(102, 204, 204), new Color(218, 170, 0) }; Color color = colors[shape.ordinal()]; g.setColor(color); g.fillRect(x + 1, y + 1, squareWidth() - 2, squareHeight() - 2); g.setColor(color.brighter()); g.drawLine(x, y + squareHeight() - 1, x, y); g.drawLine(x, y, x + squareWidth() - 1, y); g.setColor(color.darker()); g.drawLine(x + 1, y + squareHeight() - 1, x + squareWidth() - 1, y + squareHeight() - 1); g.drawLine(x + squareWidth() - 1, y + squareHeight() - 1, x + squareWidth() - 1, y + 1); } class TAdapter extends KeyAdapter { public void keyPressed(KeyEvent e) { if (!isStarted || curPiece.getShape() == Tetrominoes.NoShape) { return; } int keycode = e.getKeyCode(); if (keycode == 'p' || keycode == 'P') { pause(); return; } if (isPaused) return; switch (keycode) { case KeyEvent.VK_LEFT: tryMove(curPiece, curX - 1, curY); break; case KeyEvent.VK_RIGHT: tryMove(curPiece, curX + 1, curY); break; case KeyEvent.VK_DOWN: tryMove(curPiece.rotateRight(), curX, curY); break; case KeyEvent.VK_UP: tryMove(curPiece.rotateLeft(), curX, curY); break; case KeyEvent.VK_SPACE: dropDown(); break; case 'd': oneLineDown(); break; case 'D': oneLineDown(); break; } } } }
//Shape.java package tetris; import java.util.Random; import java.lang.Math; public class Shape { enum Tetrominoes { NoShape, ZShape, SShape, LineShape, TShape, SquareShape, LShape, MirroredLShape }; private Tetrominoes pieceShape; private int coords[][]; private int[][][] coordsTable; public Shape() { coords = new int[4][2]; setShape(Tetrominoes.NoShape); } public void setShape(Tetrominoes shape) { coordsTable = new int[][][] { { { 0, 0 }, { 0, 0 }, { 0, 0 }, { 0, 0 } }, { { 0, -1 }, { 0, 0 }, { -1, 0 }, { -1, 1 } }, { { 0, -1 }, { 0, 0 }, { 1, 0 }, { 1, 1 } }, { { 0, -1 }, { 0, 0 }, { 0, 1 }, { 0, 2 } }, { { -1, 0 }, { 0, 0 }, { 1, 0 }, { 0, 1 } }, { { 0, 0 }, { 1, 0 }, { 0, 1 }, { 1, 1 } }, { { -1, -1 }, { 0, -1 }, { 0, 0 }, { 0, 1 } }, { { 1, -1 }, { 0, -1 }, { 0, 0 }, { 0, 1 } } }; for (int i = 0; i < 4 ; i++) { for (int j = 0; j < 2; ++j) { coords[i][j] = coordsTable[shape.ordinal()][i][j]; } } pieceShape = shape; } private void setX(int index, int x) { coords[index][0] = x; } private void setY(int index, int y) { coords[index][1] = y; } public int x(int index) { return coords[index][0]; } public int y(int index) { return coords[index][1]; } public Tetrominoes getShape() { return pieceShape; } public void setRandomShape() { Random r = new Random(); int x = Math.abs(r.nextInt()) % 7 + 1; Tetrominoes[] values = Tetrominoes.values(); setShape(values[x]); } public int minX() { int m = coords[0][0]; for (int i=0; i < 4; i++) { m = Math.min(m, coords[i][0]); } return m; } public int minY() { int m = coords[0][1]; for (int i=0; i < 4; i++) { m = Math.min(m, coords[i][1]); } return m; } public Shape rotateLeft() { if (pieceShape == Tetrominoes.SquareShape) return this; Shape result = new Shape(); result.pieceShape = pieceShape; for (int i = 0; i < 4; ++i) { result.setX(i, y(i)); result.setY(i, -x(i)); } return result; } public Shape rotateRight() { if (pieceShape == Tetrominoes.SquareShape) return this; Shape result = new Shape(); result.pieceShape = pieceShape; for (int i = 0; i < 4; ++i) { result.setX(i, -y(i)); result.setY(i, x(i)); } return result; } }
//Tetris.java package tetris; import java.awt.BorderLayout; import javax.swing.JFrame; import javax.swing.JLabel; public class Tetris extends JFrame { JLabel statusbar; public Tetris() { statusbar = new JLabel(" 0"); add(statusbar, BorderLayout.SOUTH); Board board = new Board(this); add(board); board.start(); setSize(200, 400); setTitle("Tetris"); setDefaultCloseOperation(EXIT_ON_CLOSE); } public JLabel getStatusBar() { return statusbar; } public static void main(String[] args) { Tetris game = new Tetris(); game.setLocationRelativeTo(null); game.setVisible(true); } }
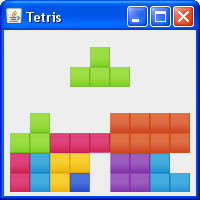
Pentru a rula codul de mai sus, apasati butonul run din Eclipse si
vi se va deschide jocul, ca-n imaginea alaturata.
Pentru a downloada proiectul cu toate fisierele faceti click aici.
Se face un proiect java, in Eclipse.
(vezi aici utilizare Eclipse pentru dezvoltarea aplicaţiilor Java)
Atentie! Numele clasei declarate public, coincide cu numele fisierului java (fara extensie)
In cadrul lui, se fac trei fisiere .java care se numesc Game, Ball si Player in care se copiaza codul de mai jos:
(vezi aici utilizare Eclipse pentru dezvoltarea aplicaţiilor Java)
Atentie! Numele clasei declarate public, coincide cu numele fisierului java (fara extensie)
In cadrul lui, se fac trei fisiere .java care se numesc Game, Ball si Player in care se copiaza codul de mai jos:
//Game.java import static java.awt.Color.green; import java.awt.Graphics; /* awt, swing = biblioteci care creeaza un proces, care se porneste cand cream un obiect JFrame; acest proces gestioneaza interfata cu utilizatorul (primeste informatii de la tastatura si mouse*/ import java.awt.Color; import java.awt.Graphics2D; import java.awt.RenderingHints; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import javax.swing.JFrame; import javax.swing.JOptionPane; import javax.swing.JPanel; @SuppressWarnings("serial") public class Game extends JPanel { //JPanel informeaza toate "ascultatoarele" care sunt inregistrate //sa primeasca notificari pt un eveniment Ball ball = new Ball(this); //instantiaza clasa minge (creeaza obiectul minge) Player player = new Player(this); //instantiaza clasa jucator (creeaza obiectul jucator) public Game() { addKeyListener(new KeyListener() { //"asculta" daca o tasta de la tastatura e apasata @Override public void keyTyped(KeyEvent e) { } @Override public void keyReleased(KeyEvent e) { player.keyReleased(e); } @Override public void keyPressed(KeyEvent e) { //se apleleaza cand e o tasta apasata player.keyPressed(e); } }); setFocusable(true); //pt a putea controla } private void move() { ball.move(); //apelam mingea player.move(); //apelam jucatorul } @Override public void paint(Graphics g) { //desenez mingea si jucatorul super.paint(g); //curata ecranul Graphics2D g2d = (Graphics2D) g; //deoarece folosesc Graphics2D, creez variabila g2d g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, //face marginile figurii (mingii) mai fina RenderingHints.VALUE_ANTIALIAS_ON); g.setColor(Color.BLUE); //mingea este de culoare albastra ball.paint(g2d); g.setColor(Color.RED); //jucatorul este de culoare rosie player.paint(g2d); setBackground(green); } public void gameOver() { //se apeleaza cand mingea atinge marginea (de jos) ferestrei JOptionPane.showMessageDialog(this, "Game Over", "Game Over", JOptionPane.YES_NO_OPTION); //afiseaza //mesaj si buton cu ok, la terminarea jocului System.exit(ABORT); } public static void main(String[] args) throws InterruptedException { JFrame frame = new JFrame("mini Tenis"); //desenam fereastra in care are loc jocul Game game = new Game(); frame.add(game); frame.setSize(300, 400); frame.setVisible(true); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //pt inchiderea jocului, o data cu fereastra while (true) { //incepe un ciclu infinit, in care apelam in mod repetat game.move(); //"move" pentru a schimba pozitia cercului game.repaint(); //apoi apelam "repaint" sa redesenam mingea in noua pozitie Thread.sleep(5); //firul curent de executie suspenda executia pt 5 milisecunde } } }
//Ball.java import java.awt.Graphics2D; import java.awt.Rectangle; public class Ball { private static final int DIAMETER = 30; //diametrul mingii int x = 0; //pozitia initiala a mingii, sus int y = 0; //pozitia initiala a mingii, stanga int xa = 1; int ya = 1; private Game game; public Ball(Game game) { this.game= game; } void move() { //fiecare if limiteaza o margine a ferestrei if (x + xa < 0) xa = 1; //mingea se deplaseaza la dreapta cu cate un pixel la fiecare runda if (x + xa > game.getWidth() - DIAMETER) //cand mingea depaseste marginea ii schimbam directia xa = -1; //mingea se deplaseaza la stanga cu cate un pixel la fiecare runda if (y + ya < 0) ya = 1; //mingea se deplaseaza in jos cu cate un pixel la fiecare runda if (y + ya > game.getHeight() - DIAMETER) //cand mingea depaseste marginea de jos a ferestrei, game.gameOver(); //terminam jocul if (collision()){ //mingea se deplaseaza in sus, daca se intersecteaza cu jucatorul ya = -1; y = game.player.getTopY() - DIAMETER; //plasam mingea deasupra jucatorului, //pt ca dreptunghiurile in care se gasesc, sa nu se intersecteze } x = x + xa; //realizeaza deplasarile de mai sus y = y + ya; //realizeaza deplasarile de mai sus } private boolean collision() { return game.player.getBounds().intersects(getBounds()); //returneaza true daca dreptunghiul //jucatorului intersecteaza dreptunghiul mingii } public void paint(Graphics2D g) { g.fillOval(x, y, DIAMETER, DIAMETER); } public Rectangle getBounds() { return new Rectangle(x, y, DIAMETER, DIAMETER); } }
//Player.java import java.awt.Graphics2D; import java.awt.Rectangle; //clasa care are o metoda de intersectie //(returneaza true cand 2 dreptunghiuri ocupa acelasi spatiu) import java.awt.event.KeyEvent; public class Player { private static final int Y = 330; //distanta jucatorului fata de marginea de sus //aceasta valoare a lui Y este folosita in metoda Paint si getBounds private static final int WIDTH = 60; //latimea paletei private static final int HEIGHT = 10; //inaltimea paletei int x = 0; //jucatorul este in partea stanga a ferestrei (coord) int xa = 0; //jucatorul este static la inceputul jocului private Game game; public Player(Game game) { this.game = game; } public void move() { if (x + xa > 0 && x + xa < game.getWidth() - WIDTH) x = x + xa; } public void paint(Graphics2D g) { g.fillRect(x, Y, WIDTH, HEIGHT); } public void keyReleased(KeyEvent e) { //cand tasta e eliberata xa = 0; //xa=o (se opreste jucatorul) } public void keyPressed(KeyEvent e) { //e.getKeyCode=obtinem codul tastei apasate //cand se apasa o tasta, se apeleaza metoda keyPressed a jucatorului if (e.getKeyCode() == KeyEvent.VK_LEFT) //cand e apasata tasta stanga xa = -2; //jucatorul se deplaseaza la stanga cu cate un pixel la fiecare runda if (e.getKeyCode() == KeyEvent.VK_RIGHT) //cand e apasata tasta dreapta xa = 2; //jucatorul se deplaseaza la dreapta cu cate un pixel la fiecare runda } public Rectangle getBounds() { //returneaza obiect de tip dreptunghi, indicand pozitia jucatorului return new Rectangle(x, Y, WIDTH, HEIGHT); //aceasta metoda va fi utilizata de catre minge, //sa cunoasca pozitia jucatorului si in acest mod sa se detecteze coliziunea } public int getTopY() { //plasam mingea deasupra jucatorului, pt ca //dreptunghiurile in care se gasesc, sa nu se interclaseze return Y; } }
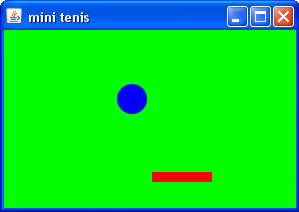
Pentru a rula codul de mai sus, apasati butonul run din Eclipse si
vi se va deschide jocul, ca-n imaginea alaturata.
Pentru a downloada proiectul cu toate fisierele faceti click aici.